nodejs+express+jade+socket.io on Ubuntu 11.04
Introduction
socket.io is a brilliant project which perfectly supports HTML5 WebSocket, in additional, it can fall back to flash or long polling when the client web browser does not support WebSocket, I explored it today on Ubuntu, I summarized the process in this post.
Install nodejs
I used nodejs v0.4.11, the latest stable version, by mannually compile/make/install.
sudo curl http://nodejs.org/dist/node-v0.4.11.tar.gz -o ~/Desktop/WayneDevLab/node.tar
sudo tar -xf node.tar cd node-v0.4.11
./configure
make
sudo make install
In the "./configure" step above if it prompted "libssl-dev" not found, please install libssl-dev (sudo apt-get install libssl-dev
) which is required by node for hosting HTTPs server.
Install npm
curl http://npmjs.org/install.sh | sh
Install express
express is an insanely fast and high performance server-side JavaScript web development framework built on node, hosting a real web server based on node will be much harder without it:)
$ npm install -g express
$ express /tmp/foo && cd /tmp/foo
$ npm install -d
Install socket.io
npm install socket.io
Setting up the Web server!!
I've modified my app.js as below:
var express = require('express');
var app = module.exports = express.createServer(),
io = require('socket.io').listen(app);
// Configuration
app.configure(function(){
app.set('views', __dirname + '/views');
app.set('view engine', 'jade');
app.set('view options', {layout: false});
app.use(express.bodyParser());
app.use(express.methodOverride());
app.use(app.router);
app.use(express.static(__dirname + '/public'));
});
app.configure('development', function(){
app.use(express.errorHandler({ dumpExceptions: true, showStack: true }));
});
app.configure('production', function(){
app.use(express.errorHandler());
});
// Routes
app.get('/', function(req, res){
res.render('index', {
title: 'Wayne Express Hello World',
youAreUsingJade: true,
domain: '192.168.1.4'
});
});
app.listen(3000);
console.log("Express server listening on port %d in %s mode", app.address().port, app.settings.env);
io.sockets.on('connection', function (socket) {
socket.emit('news', { hello: 'world' });
socket.on('my other event', function (data) {
console.log(data);
});
});
And below is my index.jade:
!!! 5 html(lang="en") head title= title script(src='http://'+ domain + ':3000/socket.io/socket.io.js') script(type='text/javascript') var socket; socket = io.connect('http://192.168.1.4:3000'); socket.on('news', function (data) { console.log(data); socket.emit('my other event', { my: 'data' }); }); body h1 Wayne WebSocket Demo using Socket.io #container - if (youAreUsingJade) p You are amazing - else p Get on it! p Powered By a(href='http://socket.io') Socket.io footer Copyright©2011 http://wayneye.com
Testing everything up
Start the server by:
$ node app.js
It works:)
I tested the combination above by using google Chromoum Dev 12 and Dev 15, they seperately adhere WebSocket protocol draft-hixie-thewebsocketprotocol-76 and draft-ietf-hybi-thewebsocketprotocol-10, the connection details for hybi10 is showing below:
socket.io will print out useful information on console when running:
Wish that helps:)
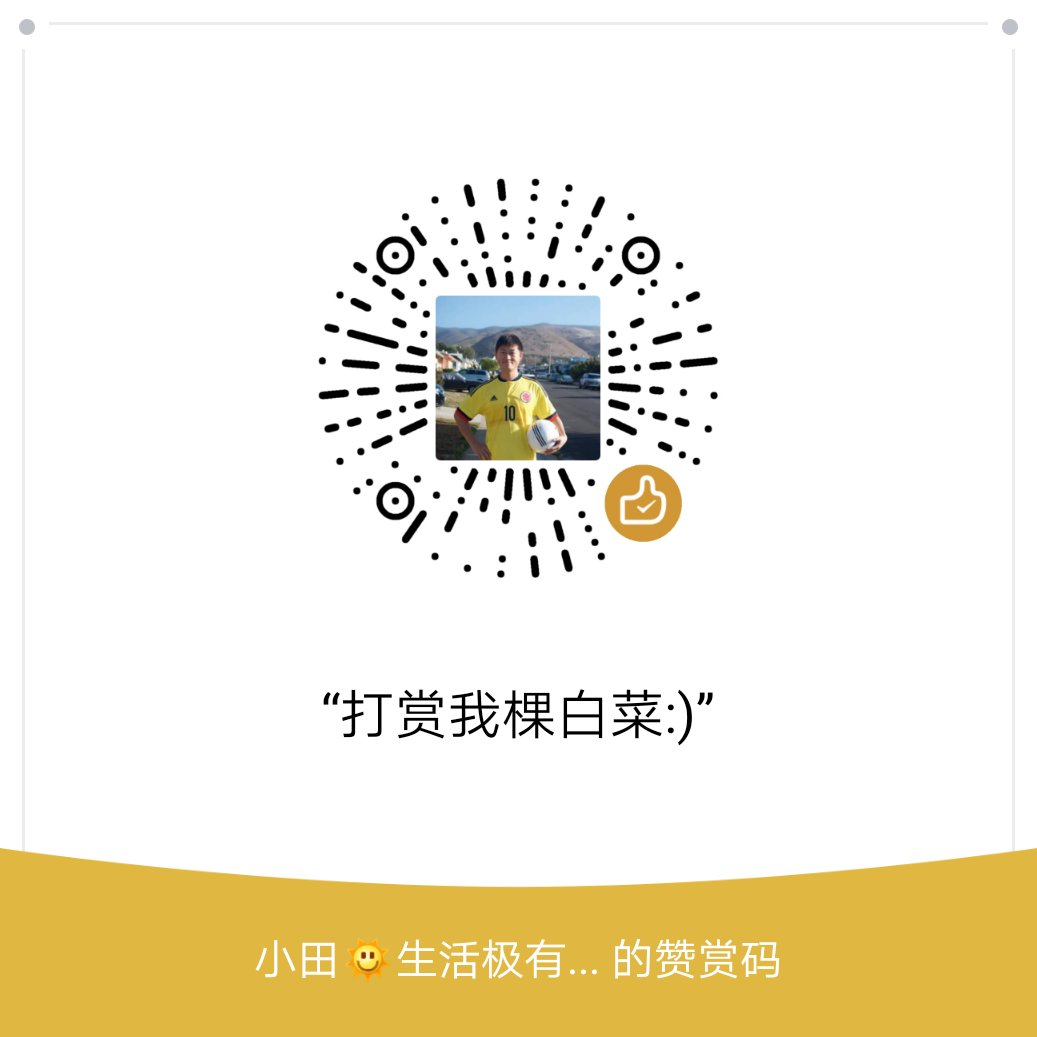
Leave a comment